C++ Variant Implementation
I did this because I needed a fast C++ implementation of base64 encoding and decoding, without any licensing problems.
C++ variant implementation. And then with std::visit we can call a visitor object that will invoke an operation based on the active type in the variant. Variant Record for Transactions. As described in the section on choosing variants, Mitsuba 2 code can be compiled into different variants, which are parameterized by their computational backend and representation of color.To enable such retargeting from a single implementation, the system relies on C++ templates and metaprogramming.
The client code in the example below passes a string to the TestObject() function, and also handles strings returned from the TestObjectReturn() function. Simple C++ functions over its component states cannot be. Sum types are objects that can vary their type dynamically.
Windows is NOT designed to be programmed using C++. Windows Implementation Libraries (WIL) The Windows Implementation Libraries (WIL) is a header-only C++ library created to make life easier for developers on Windows through readable type-safe C++ interfaces for common Windows coding patterns. DR These features are enabled in all /std compiler option modes.
Implementing a variant type from scratch in C++ October 09, 13 When programming in C++ we sometimes need heterogeneous containers that can hold more than one type of value (though not more than one at the same time). In fact, it can even rename non-variadic class templates, as in the following examples:. Bison provides a variant based implementation of semantic values for C++.
The variant class template is a safe, generic, stack-based discriminated union container, offering a simple solution for manipulating an object from a heterogeneous set of types in a uniform manner. This is where you should declare dependencies of the specific variant. In the article, we looked at a new technique to implement runtime polymorphism.
An implementation could change if it wanted to though. VBM3D is an extension to video of the well known image denoising algorithm BM3D, which takes advantage of the sparse representation of stacks of similar patches in a transform domain. First, due to the lack of support for true variadic template parameter lists in C++, the number of parameters must be limited to some implementation-defined maximum (namely, BOOST_VARIANT_LIMIT_TYPES).
MSVC has been shipping implementations of std::optional , std::any , and std::variant since the Visual Studio 17 release, but we haven’t provided any guidelines on how and when. MPark.Variant is an implementation of C++17 std::variant for C++11/14/17. Deleting the first node in the list and deleting the last node in the list.
Notable features of boost::variant include:. Some things that WIL includes to whet your appetite:. If our container needs only contain basic types and Plain Old Data Structures, the solution is simple:.
In 15, I wrote an article titled Variant Visitation which described an implementation strategy for std::visit.The approach involved a matrix of function pointers, and many have raised concerns regarding poor code-gen caused by optimization limitations of function pointers on some compilers. Here is a variant record implementation of the Transaction type type PaymentType is (Cash, Check, Credit);. MainDebugImplementation and mainReleaseImplementation) extends implementation Used for declaring implementation dependencies for a specific variant of the main component.
Sliepen's answer mentioned, leaving only recursively transforming operation for recursive_transform() may be a better idea. To get and set the string object whose content is present in stream. To enable variant-based semantic values, set the %define variable api.value.type to variant (see section %define.
When the number of variants is zero or one, the invocation of the callable object is implemented in constant time, i.e. 6 Jan • Thibaud Ehret • Pablo Arias. A variant is permitted to hold the same type more than once, and to hold differently cv-qualified versions of the.
It is almost never empty:. Variants guarantee no dynamic memory allocation (other than which is allocated by their contained types). It does not depend on sizeof(Types).
Therefore, the memory size of the input must be allocated for the sorted output to be stored in (see below for versions that need only n/2 extra spaces). C++17 adds several new “vocabulary types” – types intended to be used in the interfaces between components from different sources – to the standard library. Some implementations are released under either the GNU/GPL, or a BSD-variant, which is not what I require.
We will look at C++ projects in detail in this chapter, but most of the topics will apply to other supported native languages as well. In the following C++ implementation, we have given two methods of deletion i.e. C++17’s solution to the above problems is std::optional.
The following code example shows how to implement a covariant interface in a generic class. Mython - an extensible variant of the Python programming language, apparently a front-end for CPython. Implementing Variant Generic Interfaces.
Implementing State Machines with std::variant. Used to clear the stream. Return type deduction for functions has meant that I can drop result_type for visitors and I don’t need to rely on complex or redundant uses of decltype.
Some decent ones are available in Java, but as mentioned, I need C++ code. A simple variant type implementation in C++. Boost::variant is a C++ typesafe discriminated union;.
As mentioned as part of my answer to your previous comment, the type is not exactly known and having a huge list of if-else in code doesn't really help me as there are atleast. Resources about C++17 STL:. Using this we can read from string as if it were a stream like cin.
This alleviates all the limitations reported in the previous section, and in particular, object types can be used without pointers. With variadic templates, parameter packs and template aliases. The string stream associates a string object with a string.
Only one of the types in a variant is stored there, and in rare cases (involving exceptions while assigning and no safe way to back out) the variant can become empty. The C++ Standard committee adopted this as a retroactive Defect Report to C++11 and all later versions. However, very few people have waded into the guts of std::regex and provided a faster implementation, ABI breaking or otherwise.
It allocates a fixed portion of memory and reuses it to hold a value of one of several predefined alternative. For simplicity, and to improve readability, the examples in these tutorials will more often use this latter approach with using declarations, although note that explicit qualification is the only way to guarantee that name collisions. After a brief overview of std::variant and its usefulness, this talk will focus on the implementation of a "lambda-based in-place visitation" approach, where the user can visit a variant by simply providing a set of lambdas on the spot.
As the result, the implementation of recursive_transform function is kept in the following form. All makes it possible to have heterogeneous. Std::visit not only can take many variants, but also those variants might be of a.
Optional<T> directly addresses the issues that arise when passing or storing what may-or-may-not-currently-be an object. Continuously tested against libc++'s std::variant test suite. These are like below − clear():.
Variant is not allowed to allocate additional (dynamic) memory. C++17 In Detail by Bartek!. Optional<T> provides interfaces to determine if it contains a T and to query the stored value.
It’s OK if the overloads do not exactly. In this post, I’ll be presenting my approach and implementation of the visitation mechanism. As described in the last post, std::visit needs a function object that has overloads that accept all possible variant alternatives.
Ask Question Asked 3 years, 1 month ago. Practical C++14 and C++17 Features - by Giovanni Dicanio;. It is designed to be available to more programming languages by sticking to a more basic C style API.
C++17 std::variant for C++11/14/17. Only in corner cases where replacing its content throws and it cannot back out safely does it end up being in an empty state. This will require implementing a way of overloading arbitrary function objects.
Empty variants are also ill-formed (std::. Instantly share code, notes, and snippets. Std.variant is an implementation of variant type in D 2.0 This page was last edited on 16 August , at 06:50 (UTC).
Between the type conversion operator in C++, and the use of templates, why bother with VARIANT?. If you don’t have much experience with building native projects with Gradle, take a look at the C++ tutorials for step-by-step instructions on how to build various types of basic C++ projects as well as some common use cases. Starting from the definition of a "recursive variant", it shows the concept and implementation behind a `make_recursive_visitor` variadic function that can be used to create "local" visitors by using lambdas.
C++17 brings us std::variant that will enable new kinds of type-safe programming patterns by providing the ability to construct sum types.One interesting potential use of std::variant was presented by Ben Deane in his 16 CppCon talk “Using Types Effectively”.If you haven’t yet, you should go and watch the talk. Text is available under the Creative Commons Attribution-ShareAlike License;. A std::variant is similar to a union:.
-- The_Type is called the discriminant of the type type Transaction(The_Type:. C++17 STL Cookbook by Jacek Galowicz;. Then we display the contents of the list after insertion and each deletion.
None of these issues are things that the C++ committee claims to have any control over. In his talk he made use of C++17’s new sum type proposal:. Pythonect - a dataflow-oriented language adopting the basic Python expression syntax, implemented in Python and integrated with the Python.
Whereas standard containers such as std::vector may be thought of as "multi-value, single type," variant is "multi-type, single value.". PaymentType := Cash) is record Amount:. The Stringstream has different methods.
Here we will see the string stream in C++. A virtual function is a member function which is declared within a base class and is re-defined(Overriden) by a derived class. While convenient for typical uses, the variant class template's variadic template parameter list is limiting in two significant dimensions.
When the C++ implementation is complete, a new /std:c++ compiler option will be added, under which these features will also be available. Active 3 years, 1 month ago. Variants of merge sort are primarily concerned with reducing the space complexity and the cost of copying.
While the design is still not final, I’ve been experimenting to deliver a reference implementation. To declare an array, define the variable type, specify the name of the array followed by square brackets and specify the number of elements it should store:. When Check => CheckNumber:.
Main Variant Implementation (e.g. When you refer to a derived class object using a pointer or a reference to the base class, you can call a virtual function for that object and execute the derived class’s version of the function. A variant is not permitted to hold references, arrays, or the type void.
A list is represented as a Variant as well, just that it stores a list 'properties';. The variant requires C++14, which has made the implementation significantly easier compared to C++11. HRESULT TestObject (const _variant_t & o);.
Monostate > can be used instead). Having written about std::variant and std::visit last week, it’s time to string together some modern C++ features to build a naive basic implementation of overload, a proposed C++ feature. Variant is a replacement for raw union use.
The use of variant types provides support for polymorphism while maintaining value semantics. Sum types are objects that can vary their type dynamically. And demonstrate various C++11 implementation techniques in the process.
Variant implementation in C++. Like list of std::pair<String, Variant> (but not exactly in my implementation). It is type-safe and knows what type it is, and it carefully constructs and destroys the objects within it when it should.
The article addresses the problem of lambda-based `std::variant` and `boost::variant` recursive visitation. Peter Dimov, 26.05.15. Based on my implementation of std::variant for libc++;.
C++ Fundamentals Including C++ 17 by Kate Gregory;. Viewed 852 times 2 \$\begingroup\$ I've been. C++17 - The Complete Guide by Nicolai Josuttis;.
This is the follow-up question for A recursive_transform Function For Various Type Nested Iterable With std::variant Implementation in C++.As G. The C++ methods in the wrapper ITest class look like this:. Basically, only functions that probe or extract their current type state and value.
If the number of variants is larger than 1, the invocation of the callable object has no complexity requirements. Nim - statically typed, compiles to C, C++, and JS, features parameterised types, macros, and so on. Merge sort's most common implementation does not sort in place;.
Variant (once there is such a thing) and it will happily oblige. Variant reached a design consensus at the fall ISO C++ committee meeting in Kona, HI, USA. We first create a list by adding nodes to the head.
With std::variant we can express an object that might have many different types - like a type-safe union, all with value semantics. When Credit => CardNumber:. Implementation of the VBM3D Video Denoising Method and Some Variants.
Hoytech on Sept 14, 17 I looked into this recently, and this is the implementation I ended up choosing:. Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. Case The_Type is when Cash => Discount:.
C++ being the important part of this sentence. Both ways of accessing the elements of the std namespace (explicit qualification and using declarations) are valid in C++ and produce the exact same behavior. The proposed "default" way to visit std::variant is honestly pretty clear and simple in comparison to most C++ code.
The single-header branch. There are only a few intrinsic functions that can be applied directly to an std::variant instance in C++;.
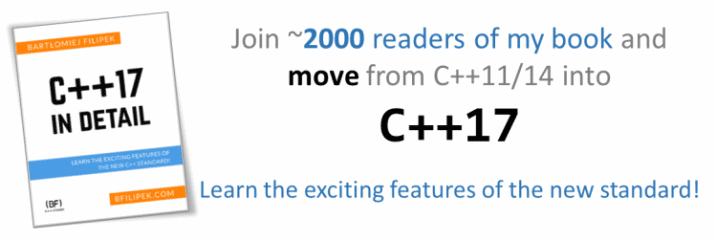
Bartek S Coding Blog Runtime Polymorphism With Std Variant And Std Visit

Std Variant Is Everything Cool About D The D Blog
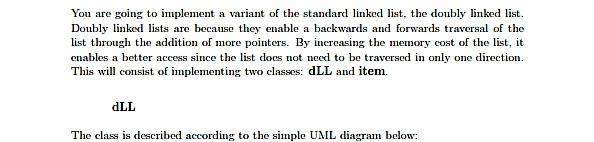
In C Use Doubly Linked List To Write The Followi Chegg Com
C++ Variant Implementation のギャラリー

C Idioms Info
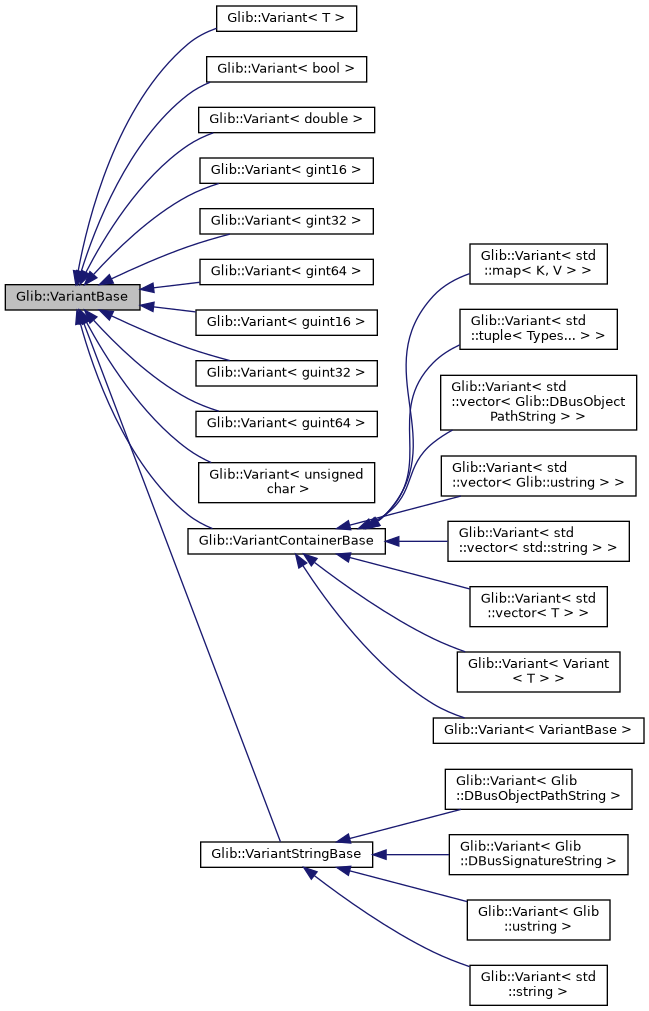
Glibmm Glib Variantbase Class Reference

Scripting The Variant Manager Setup Unreal Engine Documentation
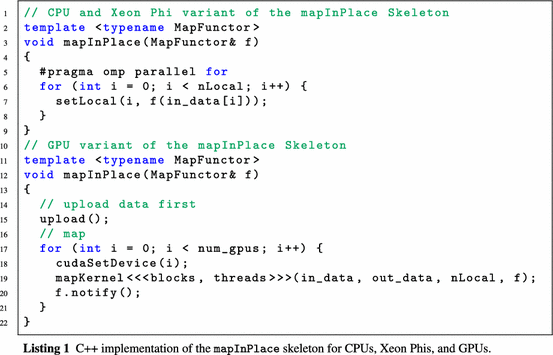
Data Parallel Algorithmic Skeletons With Accelerator Support Springerlink

History Boost Outcome Documentation 1 70 0
1

Using Variant s Qt 3d Studio 2 8 0

C 17 Rangarajan Krishnamoorthy On Programming And Other Topics

Template Subsystem Containing Subsystem Blocks Or Model Blocks As Variant Choices Simulink
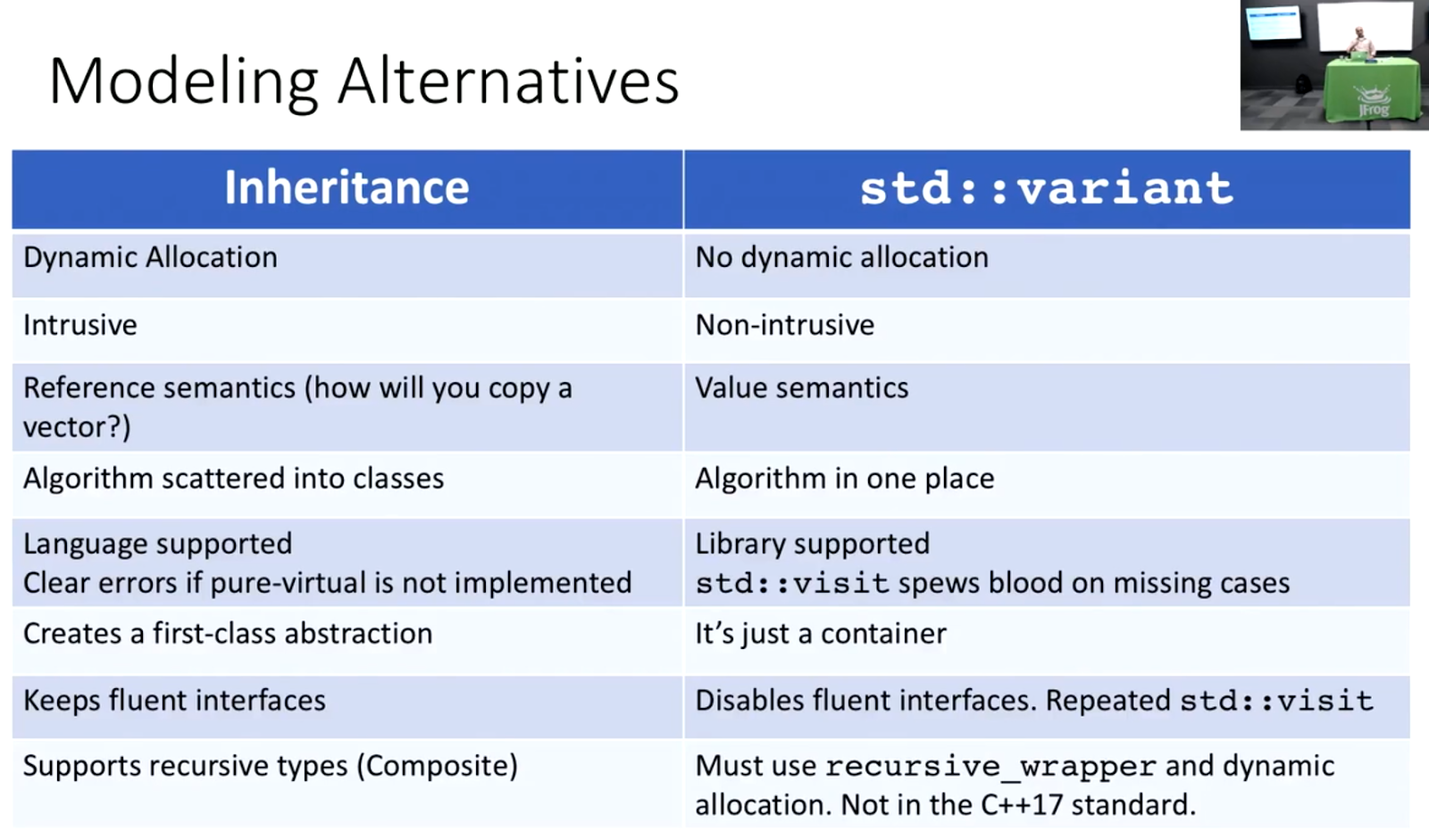
The Video Of New Tools For A More Functional C
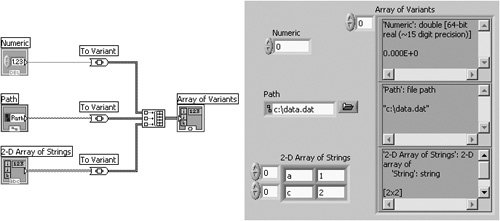
You Can Be Anything Variants Labview For Everyone Graphical Programming Made Easy And Fun 3rd Edition
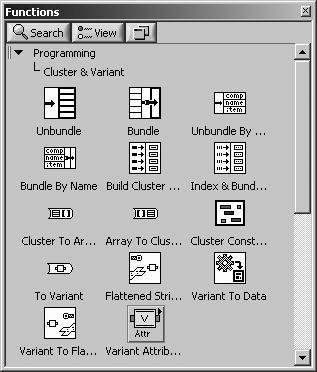
You Can Be Anything Variants Labview For Everyone Graphical Programming Made Easy And Fun 3rd Edition

Using Variant s Qt 3d Studio 2 8 0
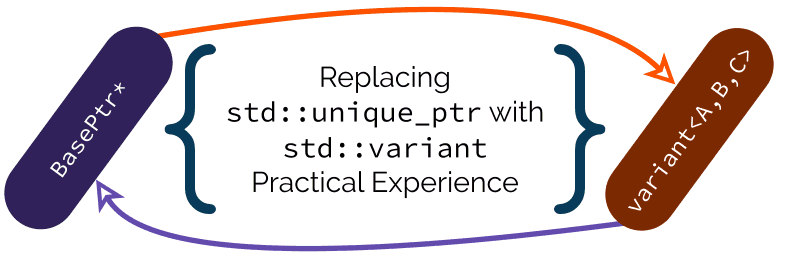
Replacing Unique Ptr With C 17 S Std Variant A Practical Experiment Dzone Iot

Implementing The Automation Technology In The Windows Applications Using Mfc Class Library And C Codes

Calling Functions On Variant Activation Unreal Engine Documentation
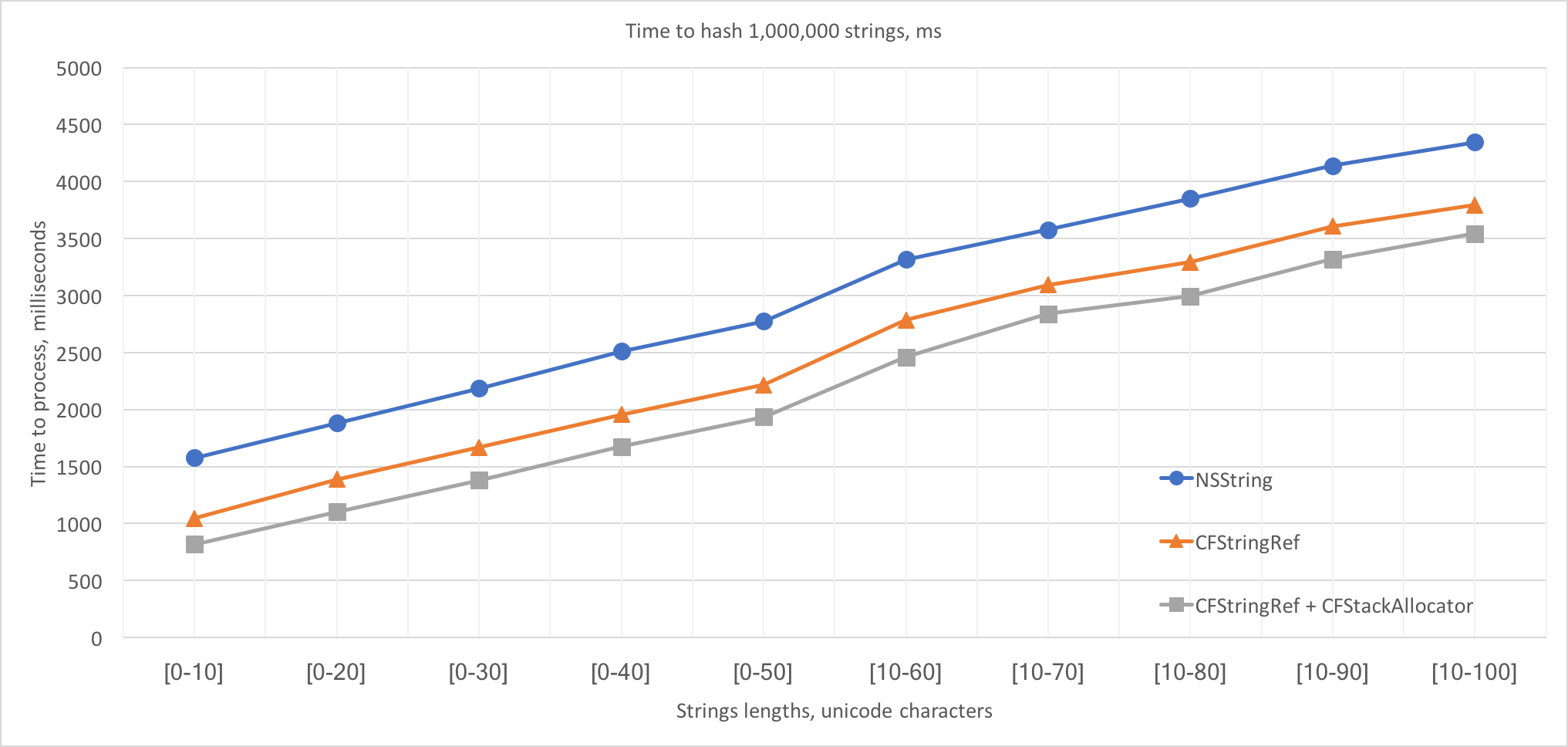
C Page 2 Michael Kazakov S Quiet Corner
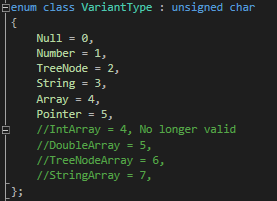
The Proper Way To Check Variant For Null Detect Nodes Without Data Flexsim Community
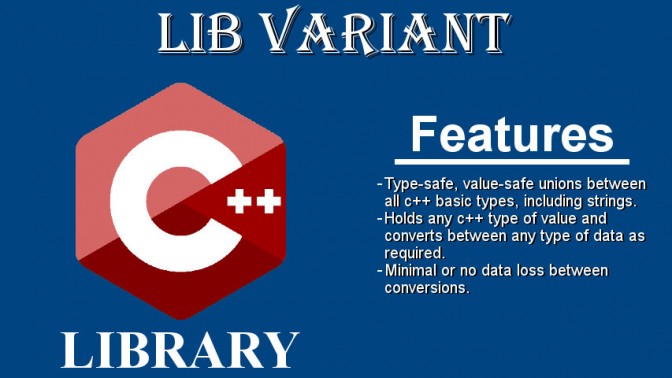
Libvariant A C Library Which Encapsulate Any Value Of Variant Types End2end Zone

A Guided Tour Of The Poco C Libraries
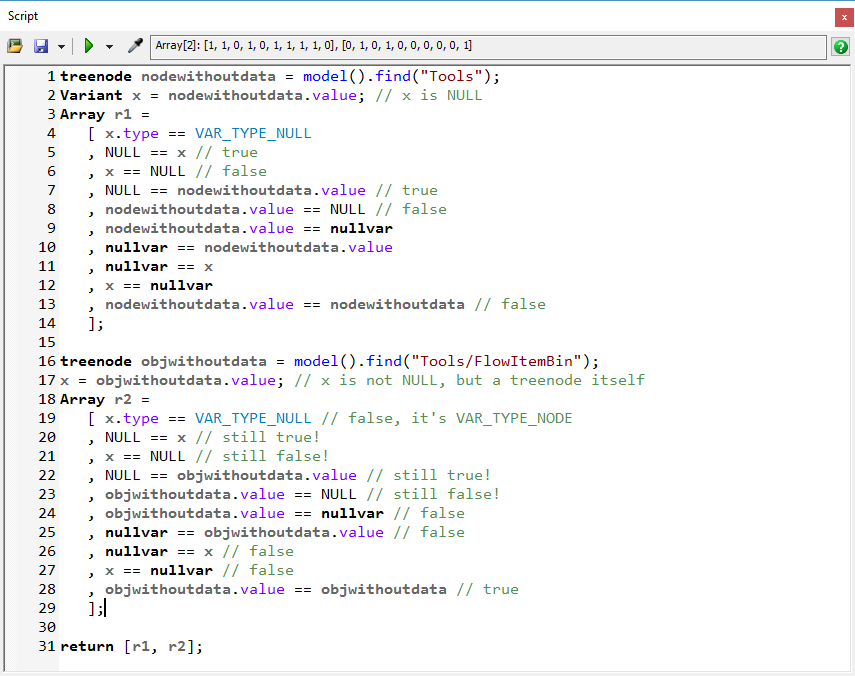
The Proper Way To Check Variant For Null Detect Nodes Without Data Flexsim Community
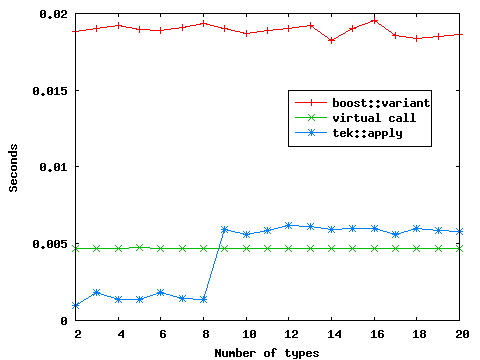
High Performance Heterogeneous Container Codeproject
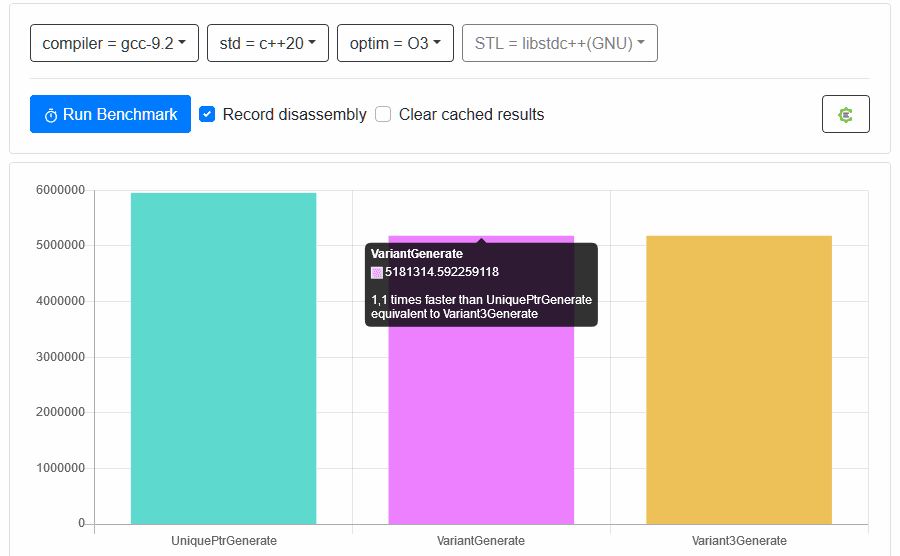
Bartek S Coding Blog Runtime Polymorphism With Std Variant And Std Visit
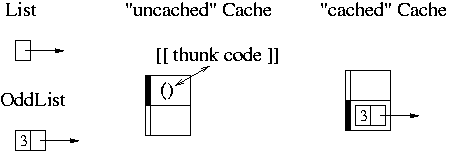
Fc Lazy List Implementation
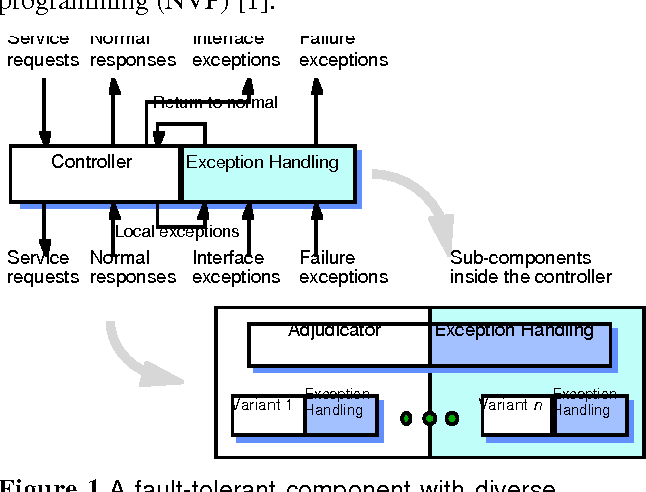
Pdf Implementing Software Fault Tolerance In C And Open C An Object Oriented And Reflective Approach Semantic Scholar
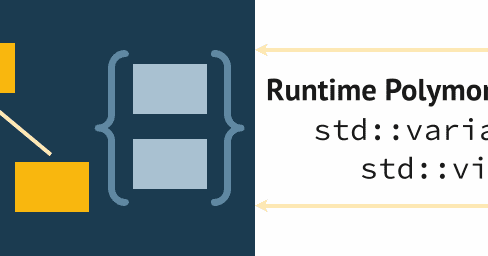
Bartek S Coding Blog Runtime Polymorphism With Std Variant And Std Visit
Managing Genomic Variant Calling Workflows With Swift T

Std Variant Vs Inheritance Vs Other Ways Performance Stack Overflow
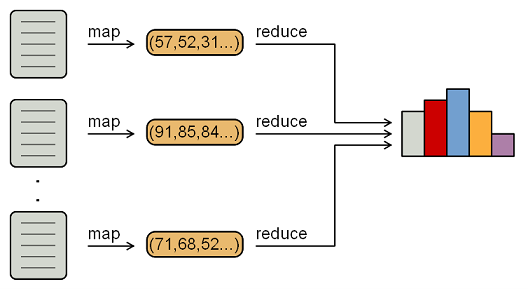
Distribution Of Population Age With Qtconcurrent Mappedreduced
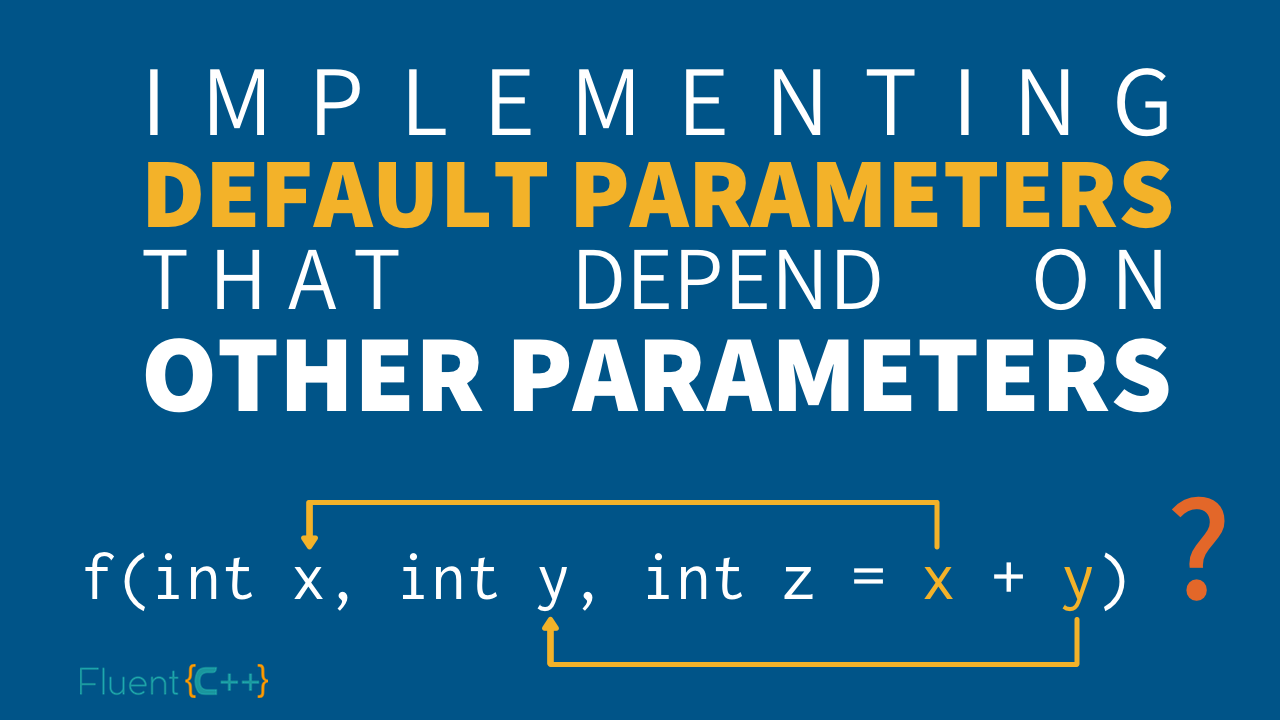
Implementing Default Parameters That Depend On Other Parameters In C Fluent C
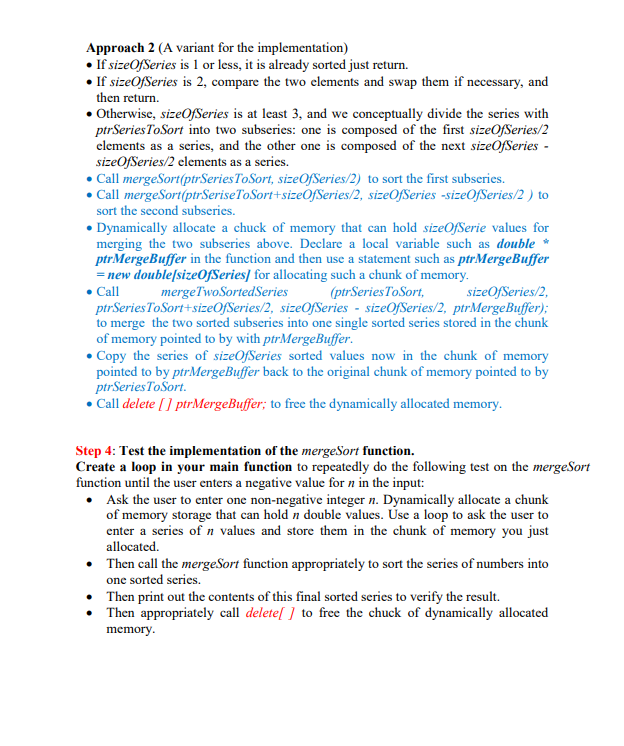
Using C Parellel Implementation Of The Merge Sor Chegg Com
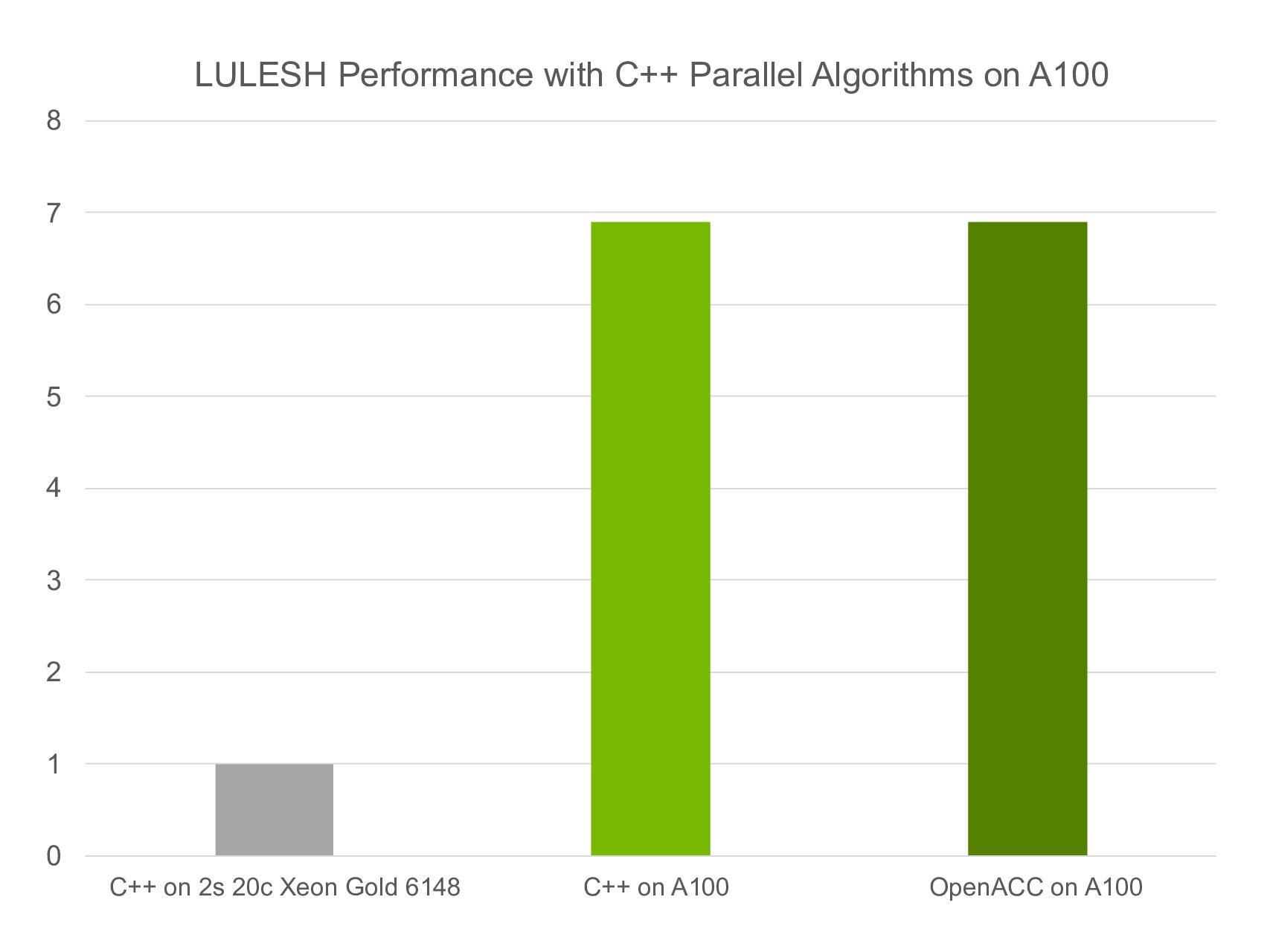
Accelerating Standard C With Gpus Using Stdpar Nvidia Developer Blog

An Efficient Variant Of The Priority Flood Algorithm For Filling Depressions In Raster Digital Elevation Models Sciencedirect
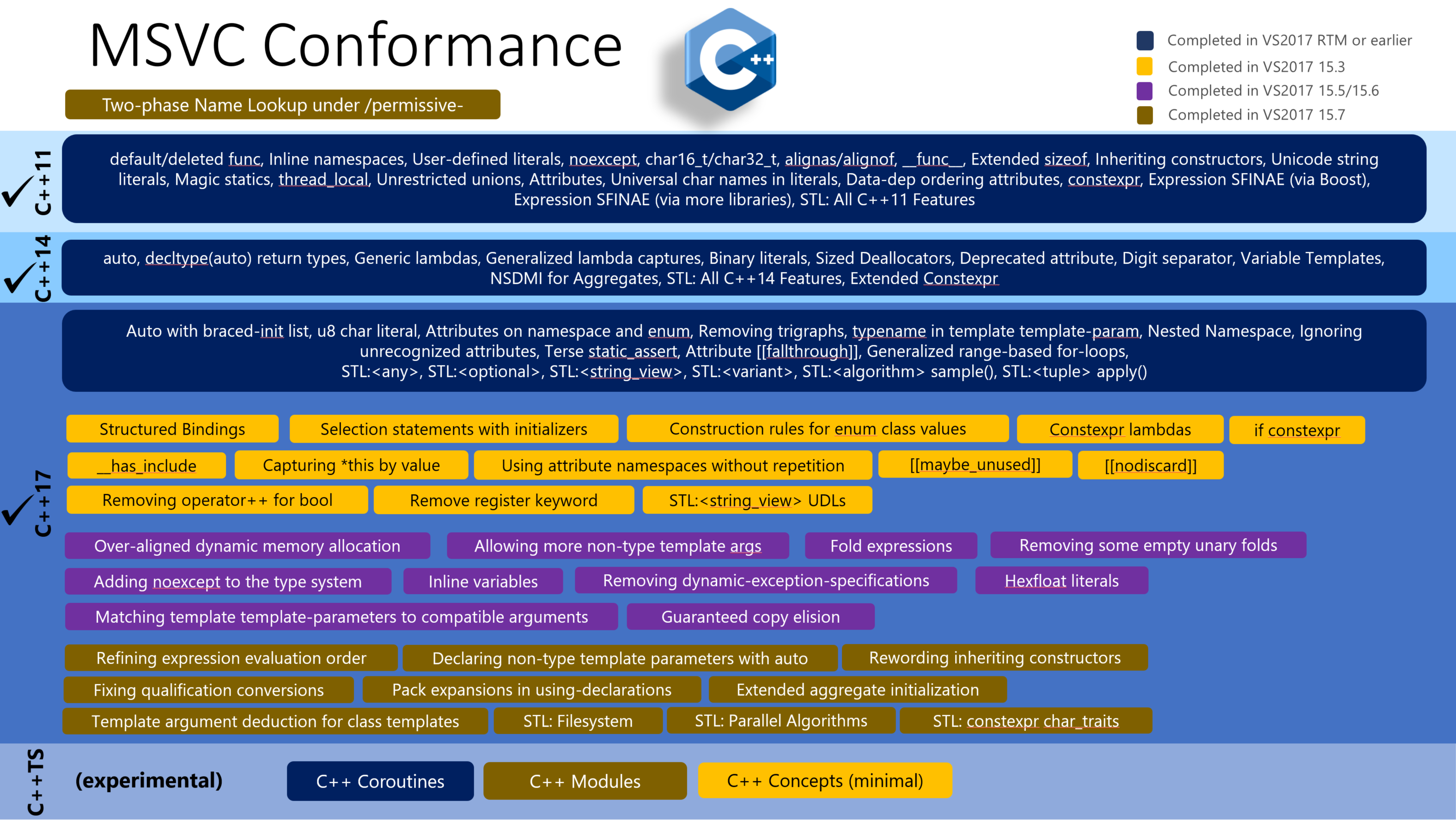
Announcing Msvc Conforms To The C Standard C Team Blog

Customize Code Generation Using Ibm Rational Rhapsody For C
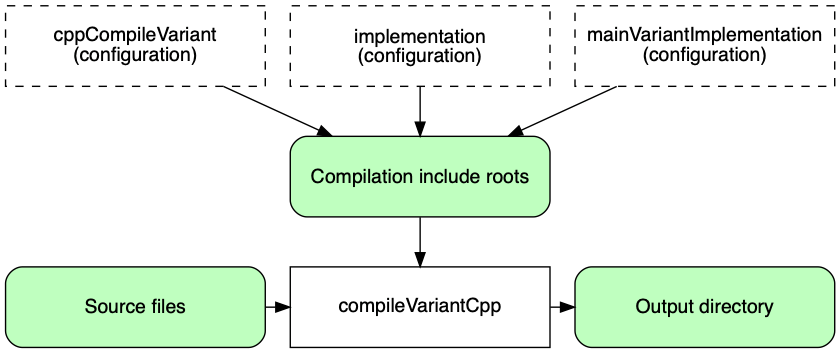
Building C Projects
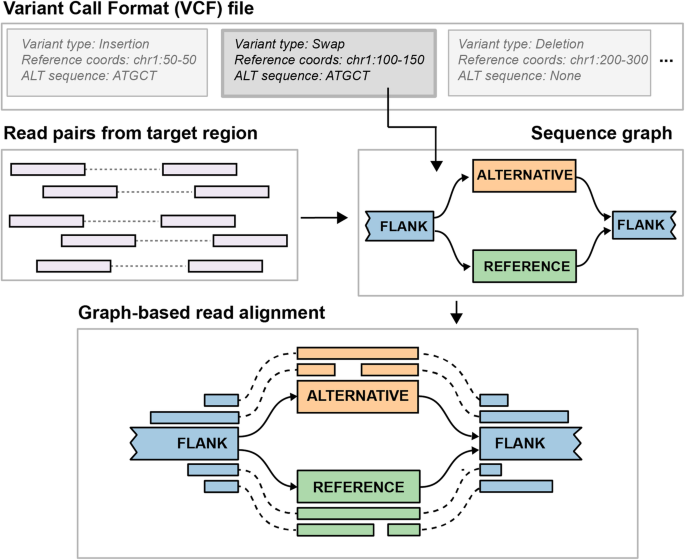
Paragraph A Graph Based Structural Variant Genotyper For Short Read Sequence Data Genome Biology Full Text

Customize Code Generation Using Ibm Rational Rhapsody For C

Bridge Pattern Wikipedia
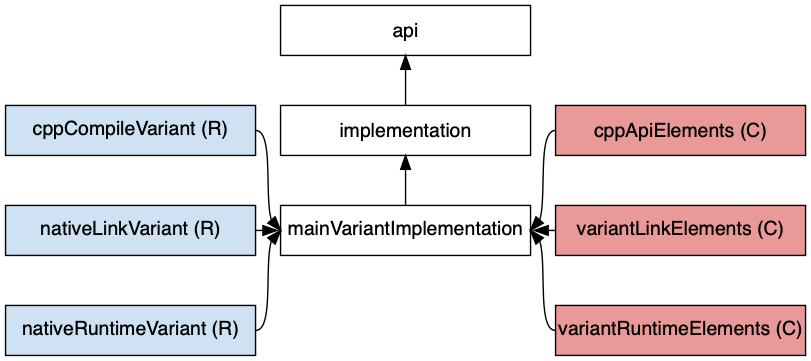
C Library

A Variant Of Recursive Decent Parsing Bjorn Fahller Lightning Talks Meeting C 17 Youtube
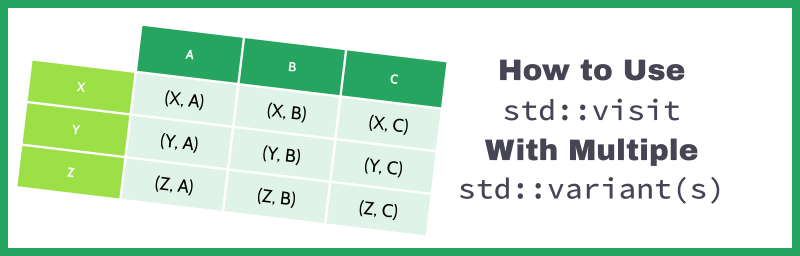
Bartek S Coding Blog How To Use Std Visit With Multiple Variants
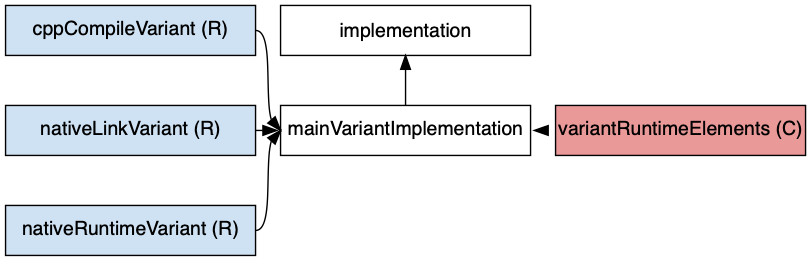
C Application

Vittorio Romeo S Website

A New Azorult C Variant Can Establish Rdp Connections Terabitweb Blog
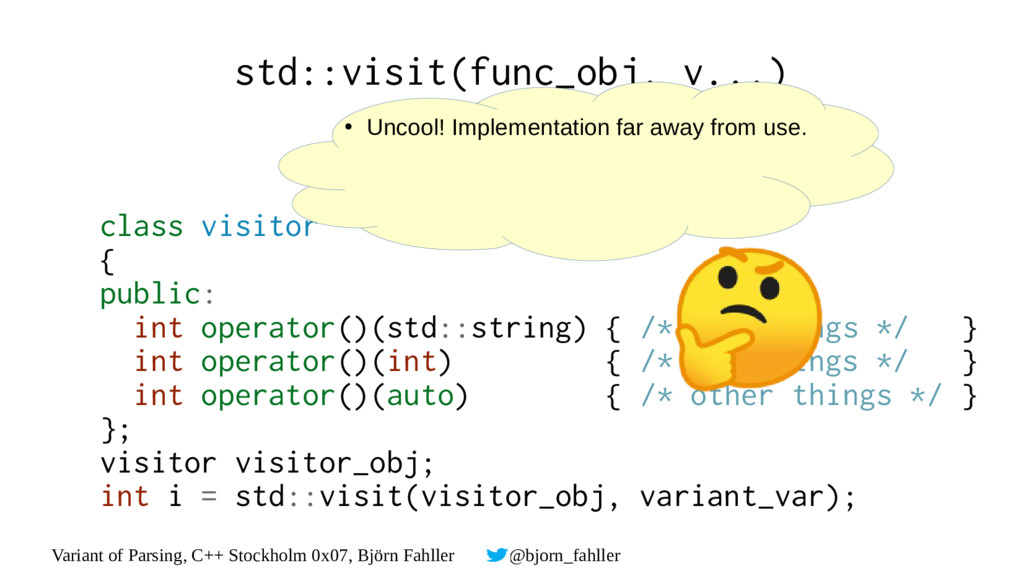
A Variant Of Recursive Descent Parsing Speaker Deck
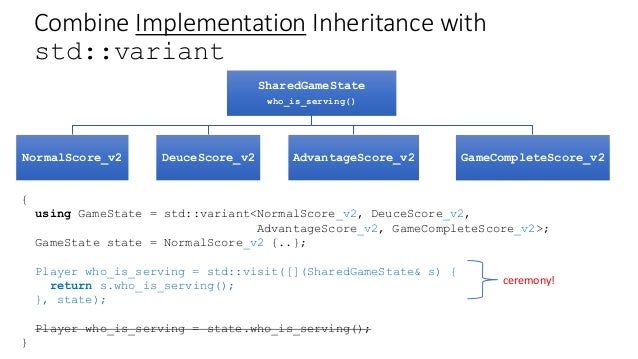
New Tools For A More Functional C

Bridge Pattern Wikipedia
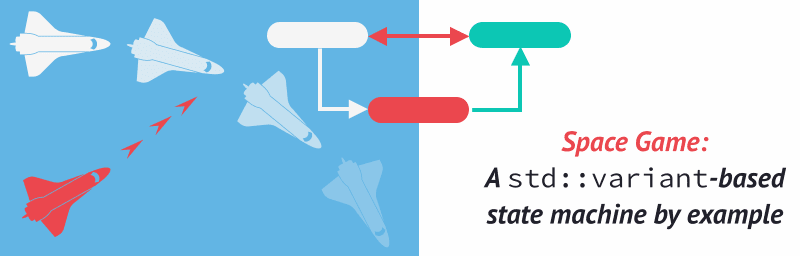
Bartek S Coding Blog Space Game A Std Variant Based State Machine By Example
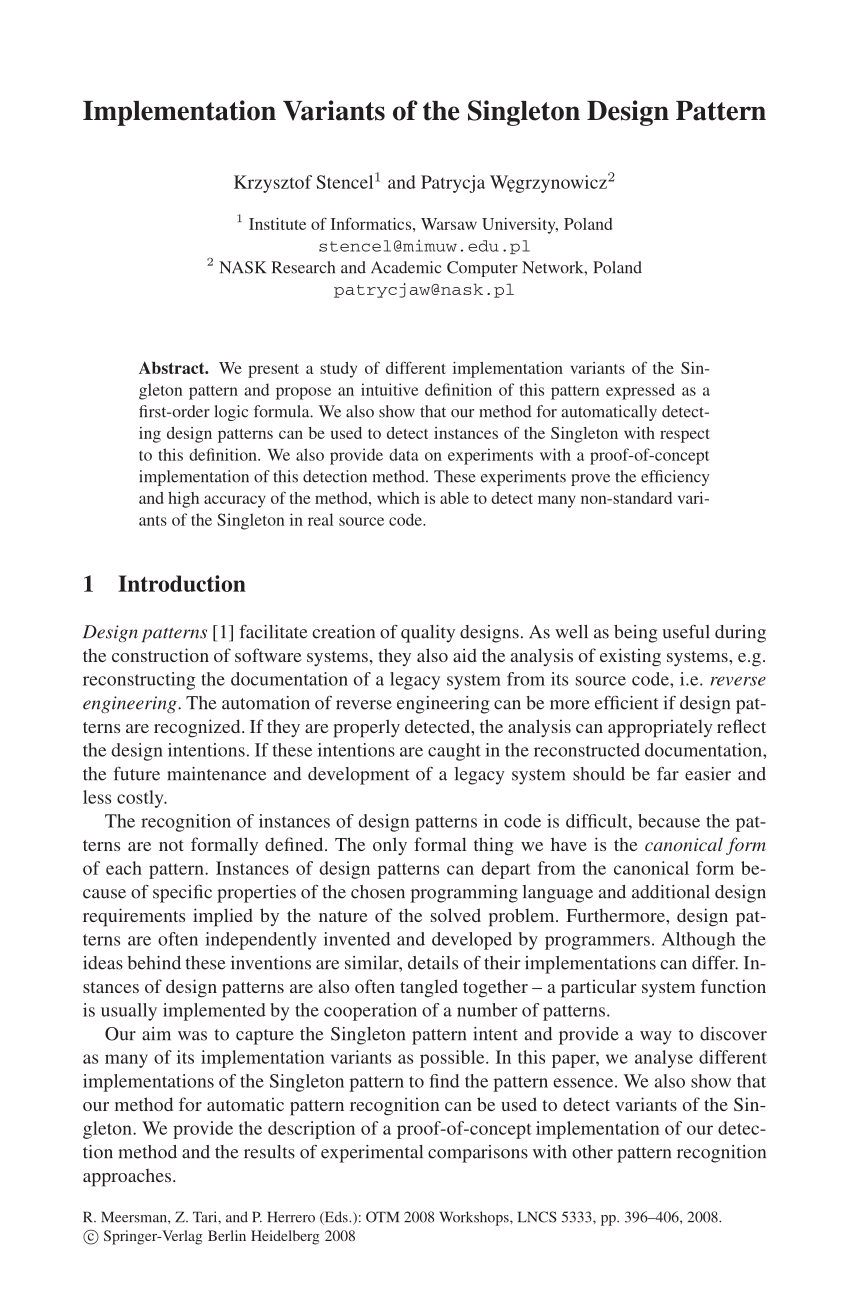
Pdf Implementation Variants Of The Singleton Design Pattern
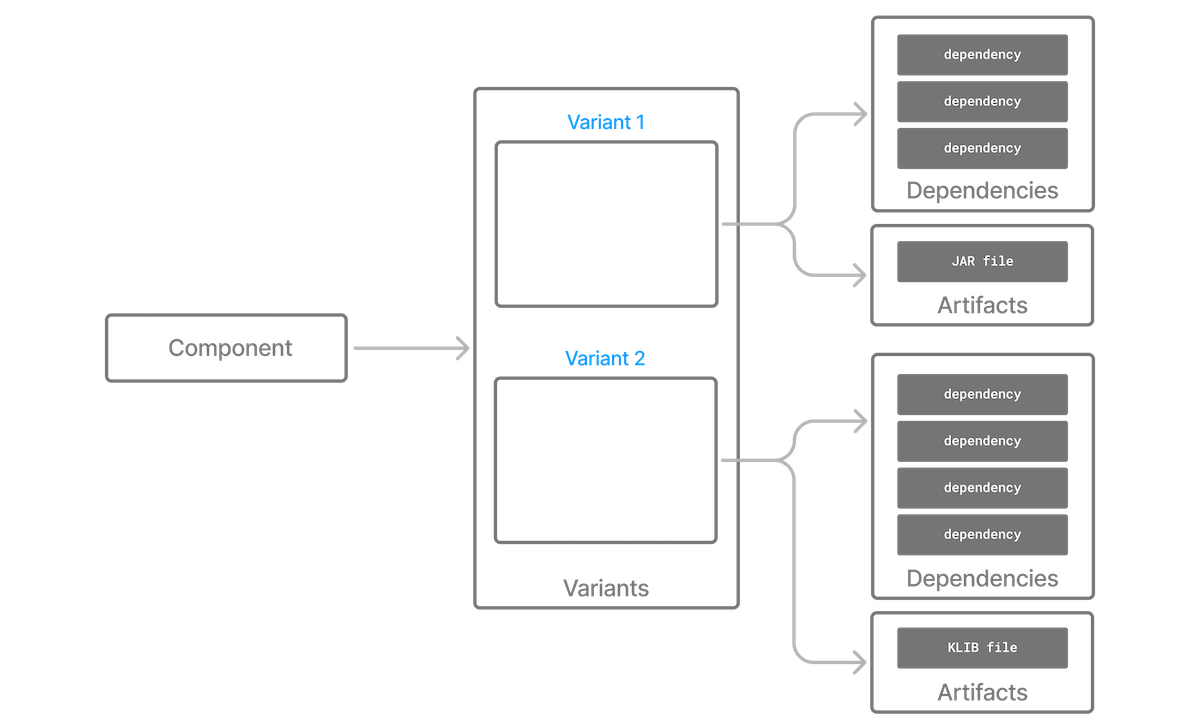
Understanding Variant Selection
Arxiv Org Pdf 1502
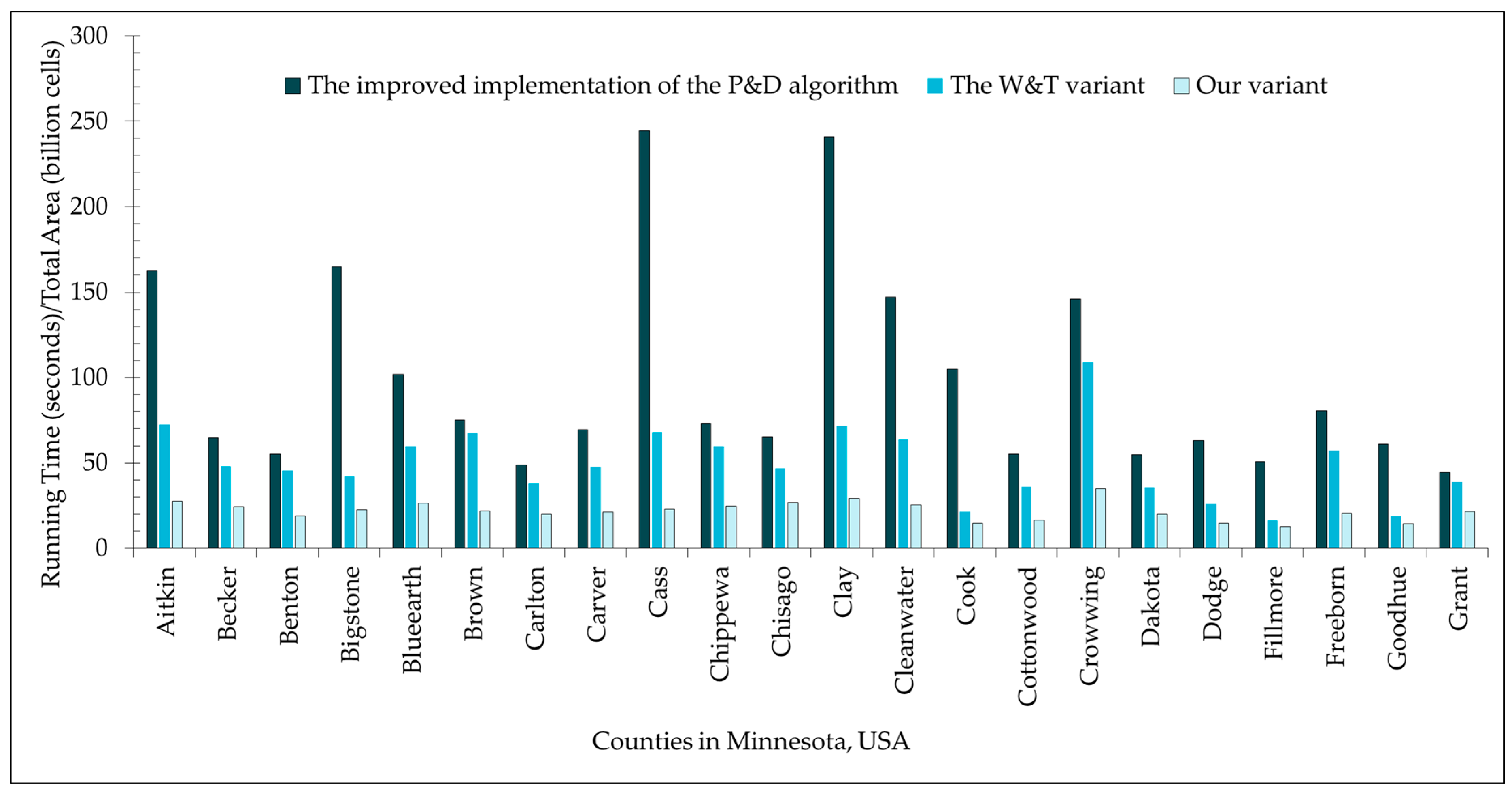
Ijgi Free Full Text A Variant Of The Planchon And Darboux Algorithm For Filling Depressions In Raster Digital Elevation Models Html
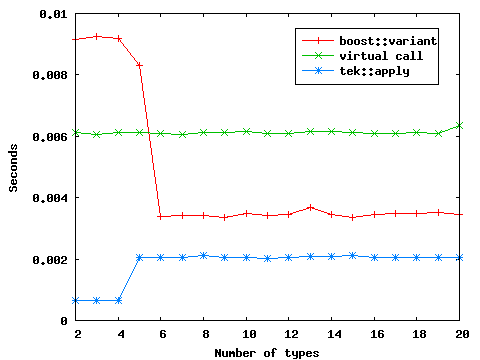
High Performance Heterogeneous Container Codeproject

Priority Queue In C Journaldev

A True Heterogeneous Container In C Andy G S Blog
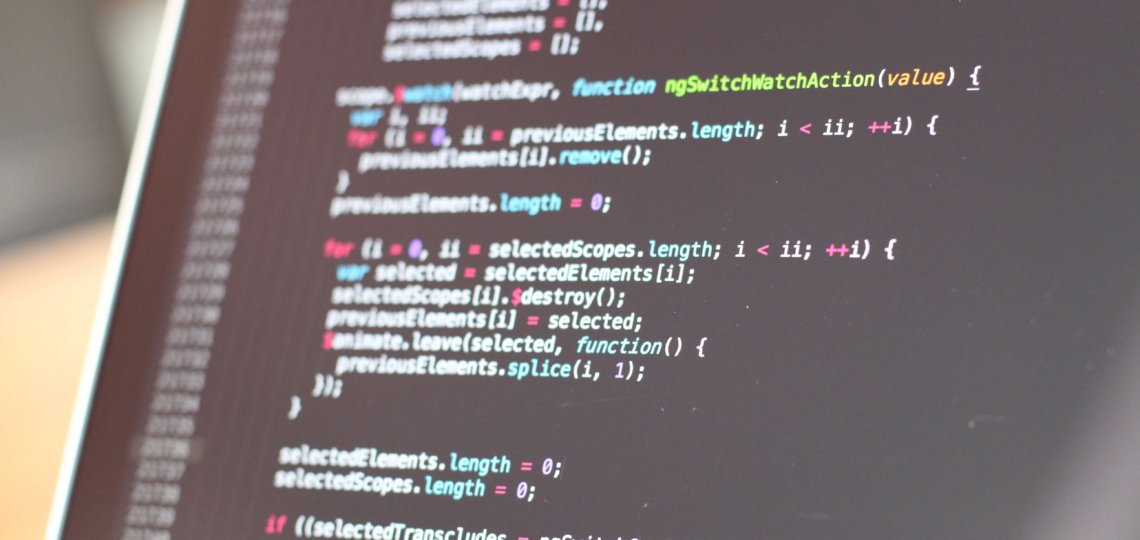
Implementing A State Machine In C 17 Blogersii

Scripting The Variant Manager Setup Unreal Engine Documentation

Example Illustrating The Handling Of Common Variants By Emulating The Download Scientific Diagram
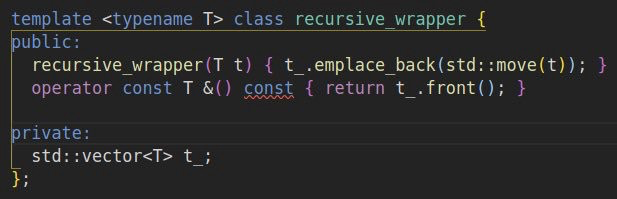
Breaking Circular Dependencies In Recursive Union Types With C 17 By Don T Compute In Public Medium

C Now 17 Vittorio Romeo Implementing Variant Visitation Using Lambdas Youtube

C Python S Map Function Modernescpp Com
Http Www Stroustrup Com Oopsla Typeswitch Draft Pdf

C Library
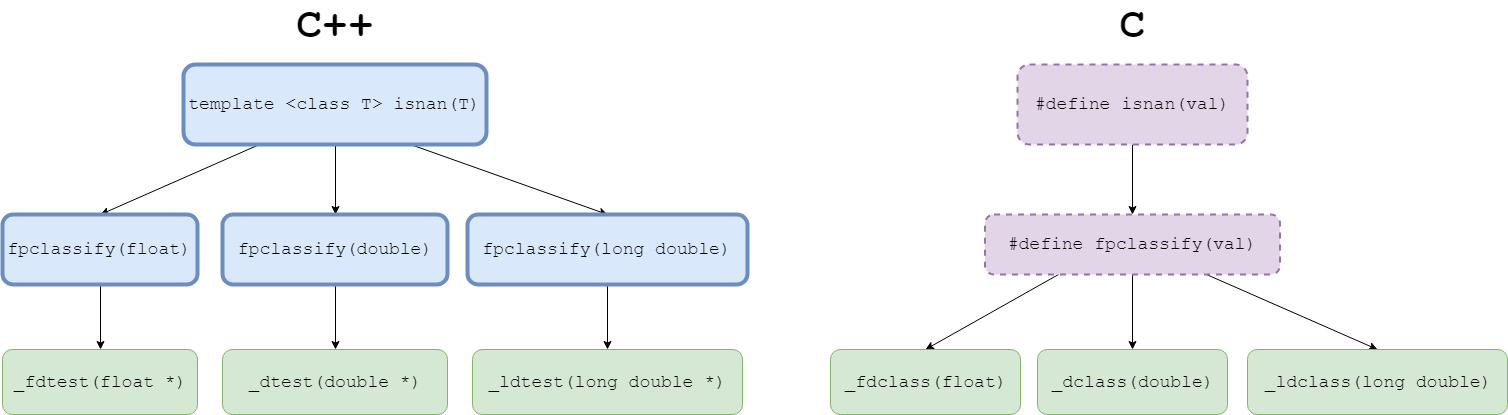
Improving The Performance Of Standard Library Functions C Team Blog
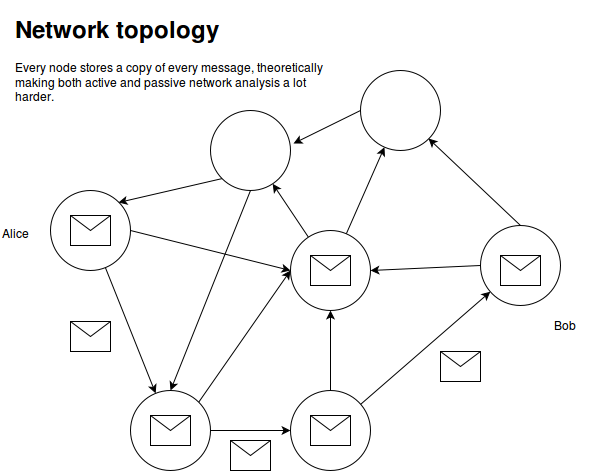
Smsg A Bitmessage Variant Market Protocol

Fpgas That Speak Your Language Cern Courier

How To Implement A Std Variant With A Recursive Std Unordered Map Cpp Questions
Algorithm Implementation Linear Algebra Tridiagonal Matrix Algorithm Wikibooks Open Books For An Open World

Shows A Variant Of The Dijkstra S Algorithm Which Is Written In C Download Scientific Diagram
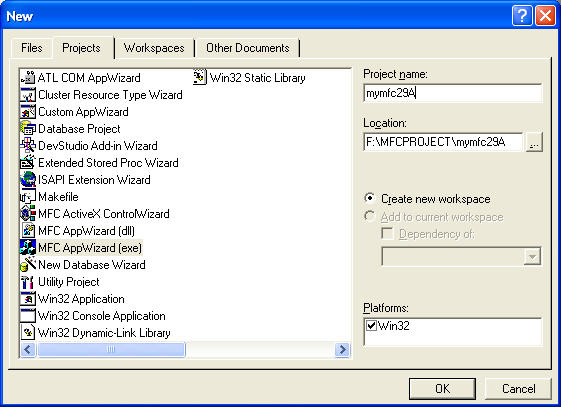
Implementing The Automation Technology In The Windows Applications Using Mfc Class Library And C Codes
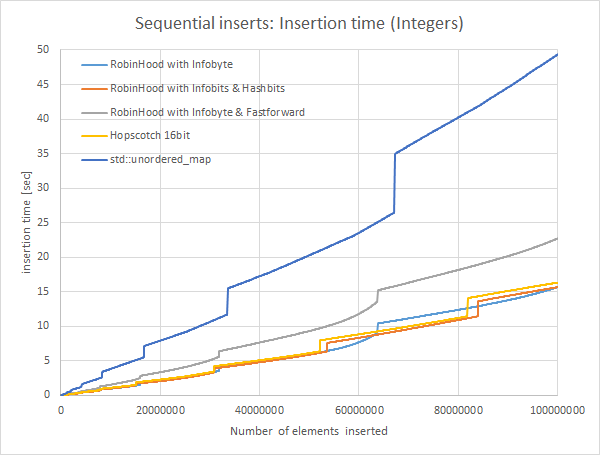
Very Fast Hashmap In C Benchmark Results Part 3

Q Tbn 3aand9gcritp1ar86tr5ugpct8xpe Efcimg Bvkxng Usqp Cau
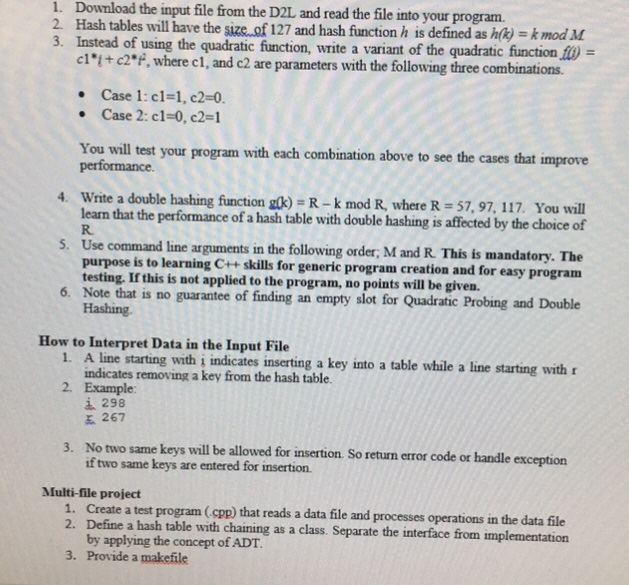
Solved I Need Help On Implementing 3 Of The Instruction Chegg Com

Interface And Implementation Classes

Stop Reimplementing The Virtual Table And Start Using Double Dispatch Andy G S Blog
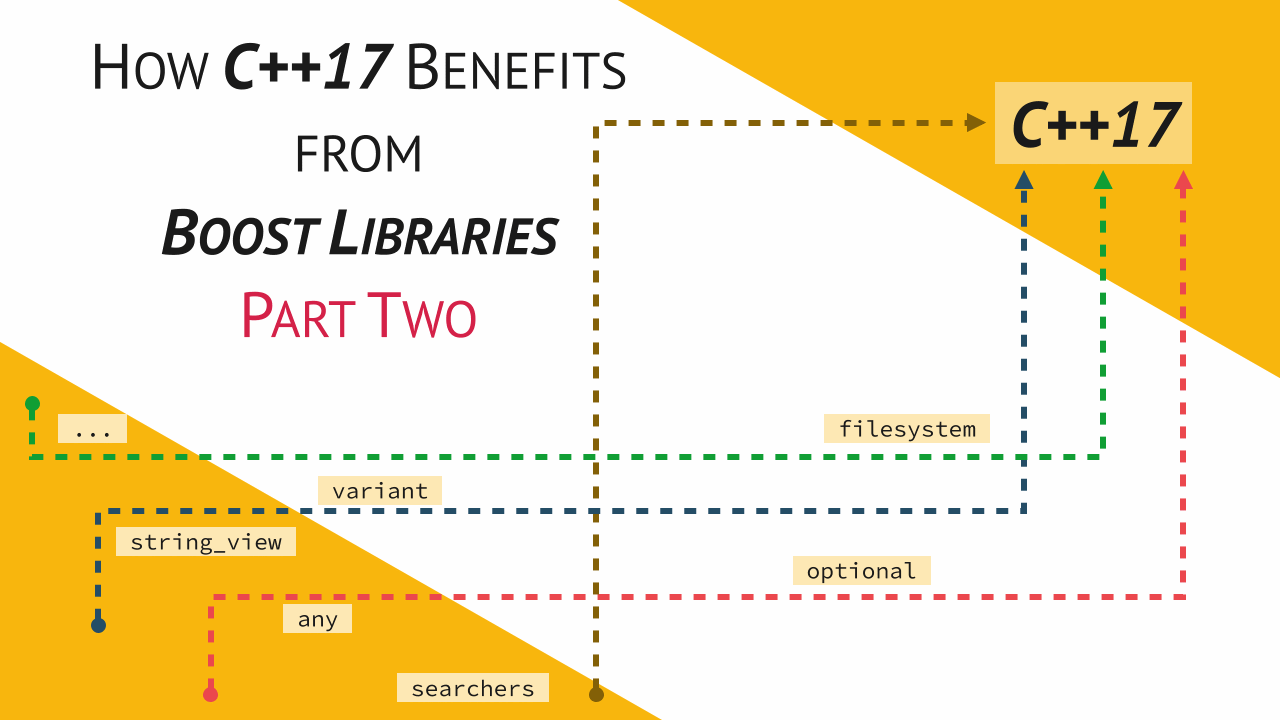
How C 17 Benefits From Boost Libraries Part Two Fluent C
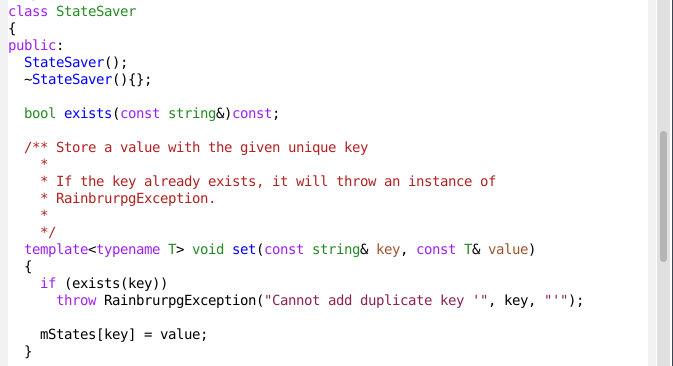
How To Make A Map Of Variant In C
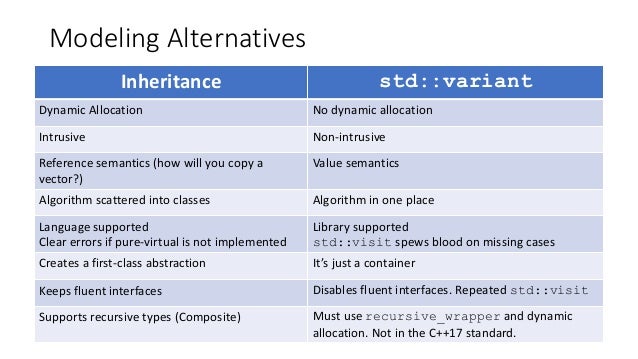
New Tools For A More Functional C

Top 25 C Api Design Mistakes And How To Avoid Them
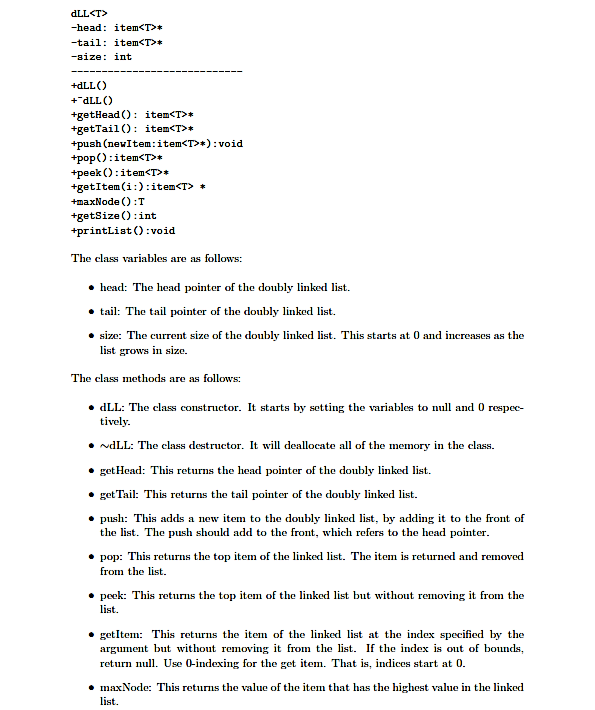
In C Use Doubly Linked List To Write The Followi Chegg Com

Std Variant Vs Inheritance Vs Other Ways Performance Stack Overflow
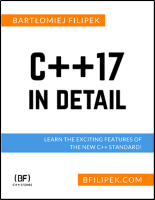
Bartek S Coding Blog Everything You Need To Know About Std Variant From C 17

Implementing A State Machine In C 17 Part 2 Blogersii

Clingen Allele Registry Links Information About Genetic Variants Pawliczek 18 Human Mutation Wiley Online Library
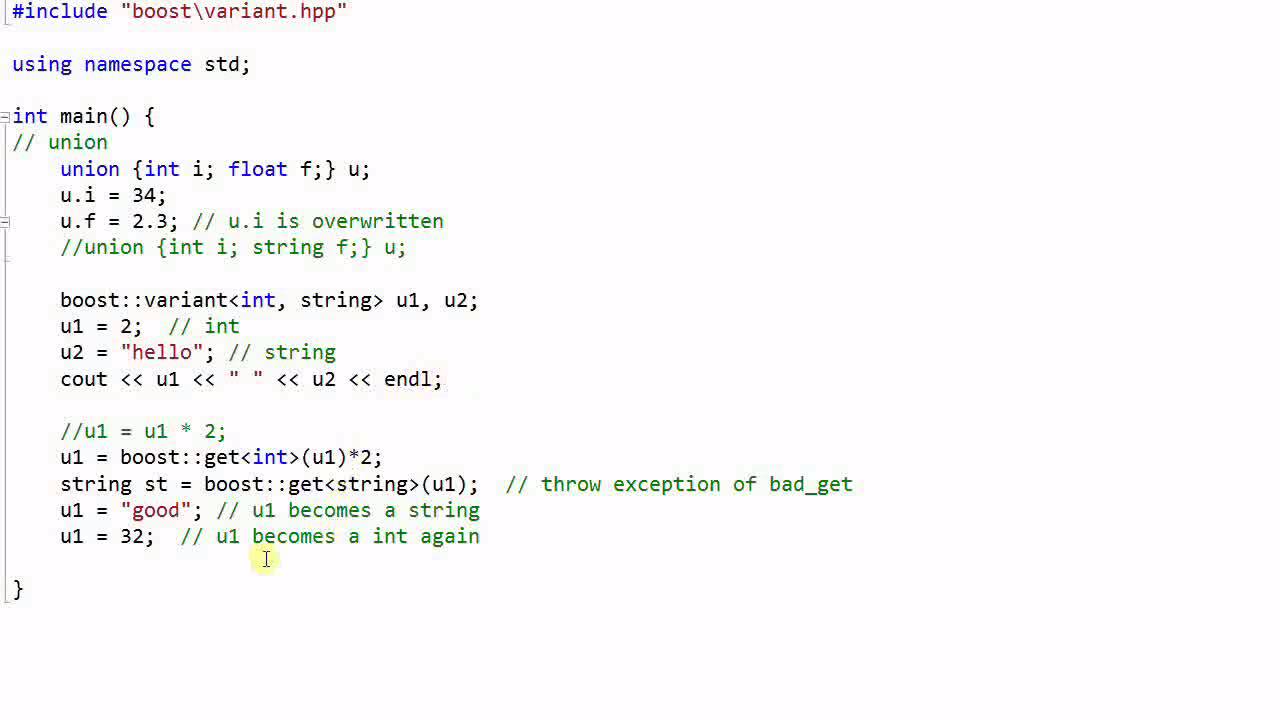
Boost Library 2 Variant Youtube
Managing Genomic Variant Calling Workflows With Swift T

C 17 Has A Visitor Modernescpp Com
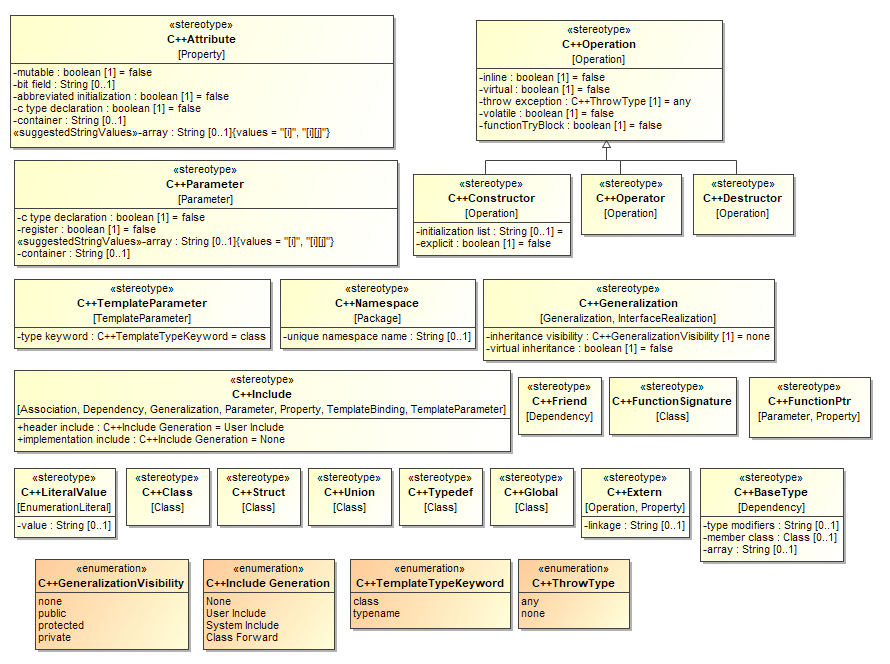
C Mapping To Uml
Fabien Pean Personal Website

Template Method Pattern Wikipedia
Q Tbn 3aand9gctbvrersnzumjnvcevp3voi017traecfb6lhes8uswi4m7y9zyl Usqp Cau
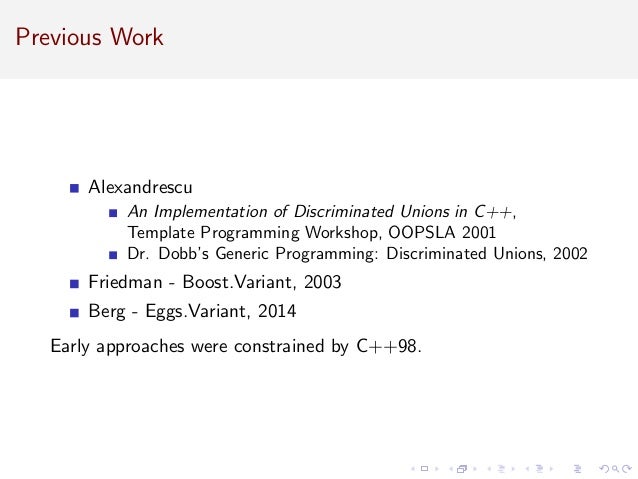
Discriminating Unions The Long Road To Std Variant

How To Make C More Real Time Friendly Embedded Com
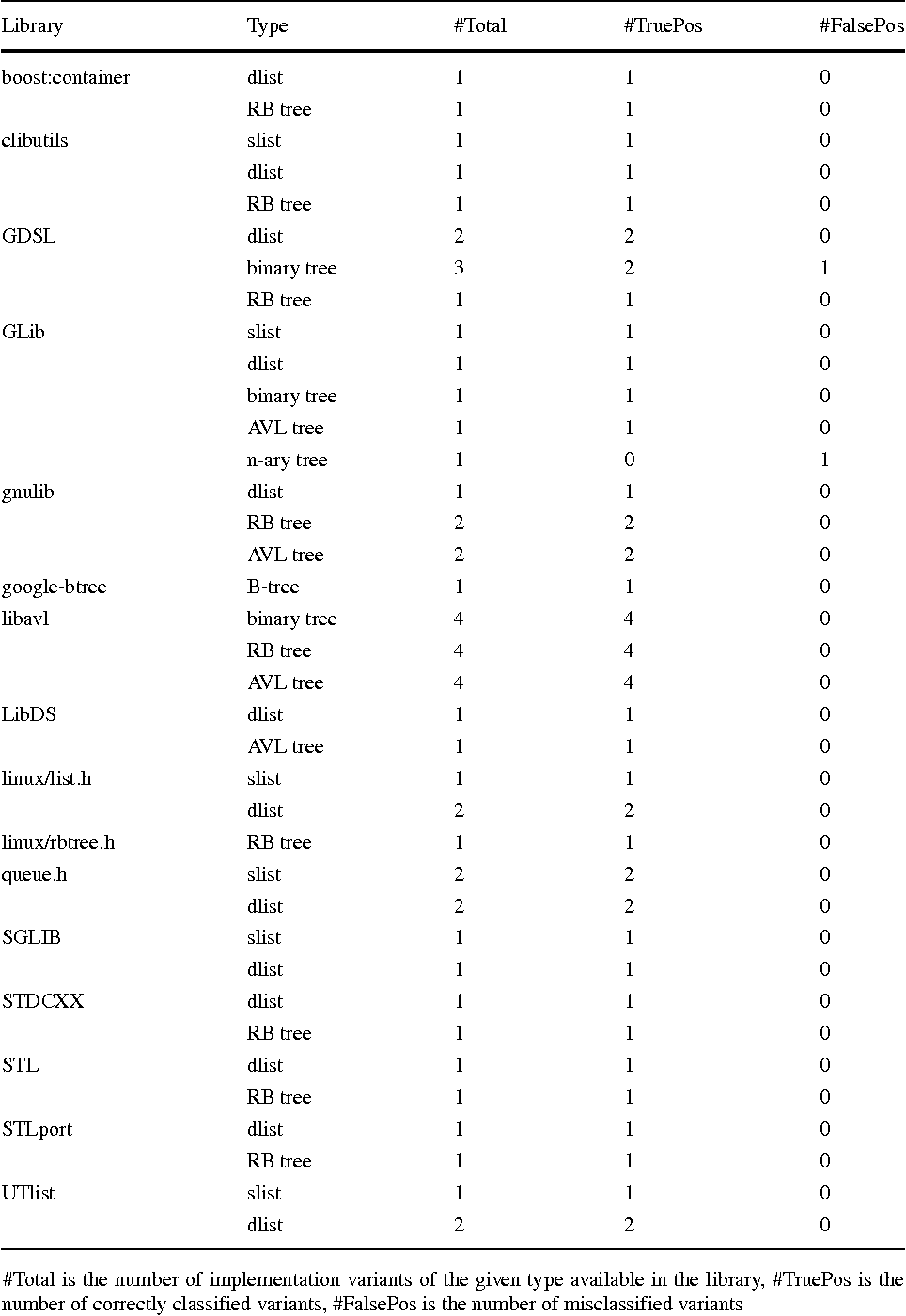
Pdf Scalable Data Structure Detection And Classification For C C Binaries Semantic Scholar
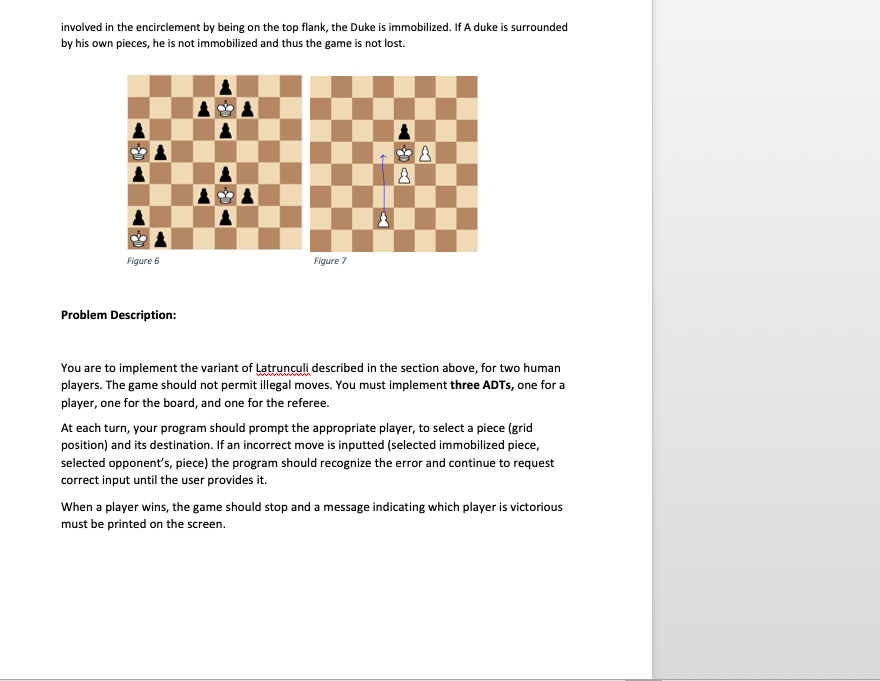
C Programming Task Please Help Me Complete This Chegg Com
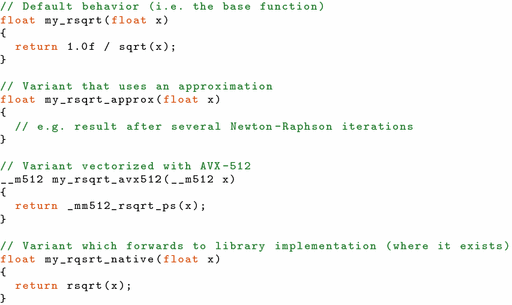
Supporting Function Variants In Openmp Springerlink
Q Tbn 3aand9gctm8xlbp74fkoh9l5btqgqhbtf8bz1rfimqscyc9i6wuyvdmvc2 Usqp Cau

C Idioms Info
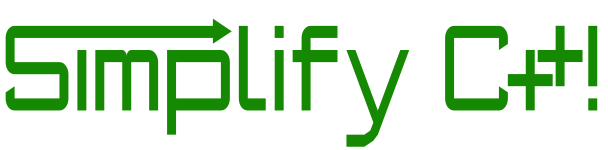
Modern C Features Std Variant And Std Visit Simplify C